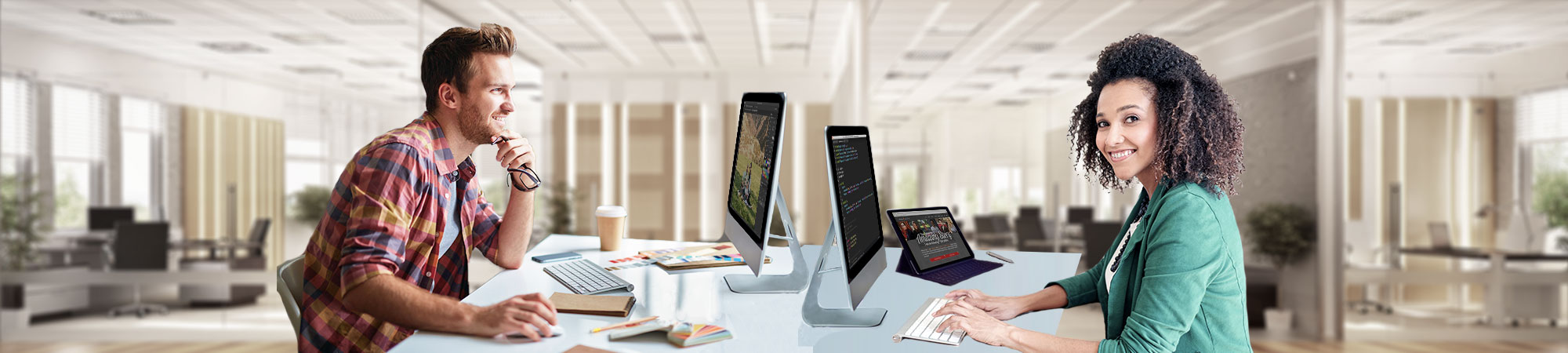
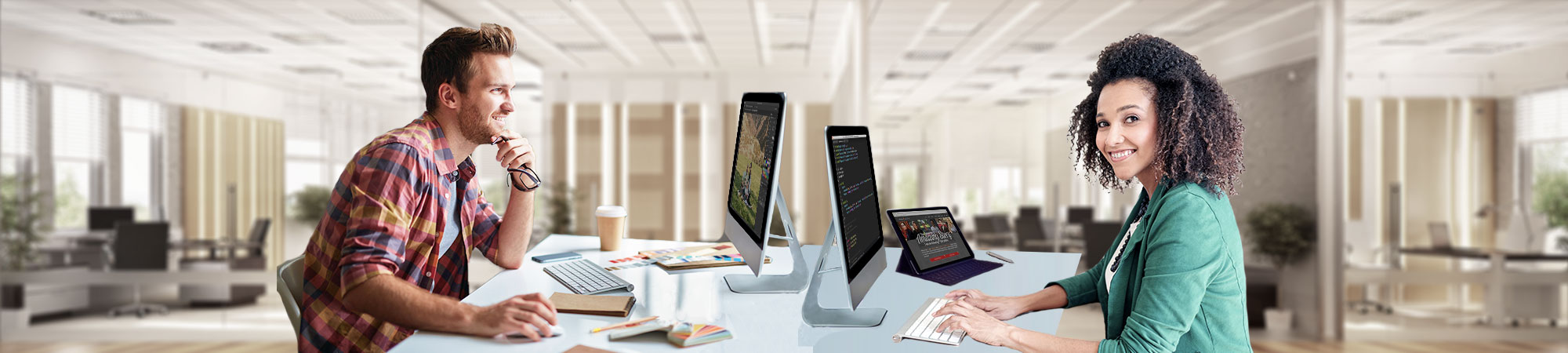
Thanks to Paul Irish
/* apply a natural box layout model to all elements */
*, *:before, *:after {
-moz-box-sizing: border-box; -webkit-box-sizing: border-box; box-sizing: border-box;
}
<meta name="viewport" content="width=device-width, initial-scale=1">
Run this command in the terminal to enable Chrome to read your local json/xml files: For Mac:
open /Applications/Google\ Chrome.app --args --allow-file-access-from-files
For Windows:
"C:\Program Files (x86)\Google\Chrome\Application\chrome.exe" --allow-file-access-from-files
<?php print get_post(7827)->post_content; ?>
With Wordpress Formatting:
<?php print apply_filters( 'the_content', (get_post(7658)->post_content) ); ?>
Simply add this to your functions.php file and jQuery will be deferred until after the page has loaded. Check Google PageSpeed to verify that you aren't getting a performance hit.
function optimize_jquery() {
if (!is_admin()) {
wp_deregister_script('jquery');
wp_deregister_script('jquery-migrate.min');
wp_deregister_script('comment-reply.min');
$protocol='http:';
if($_SERVER['HTTPS']=='on') {
$protocol='https:';
}
wp_register_script('jquery', $protocol.'https://ajax.googleapis.com/ajax/libs/jquery/1.9.0/jquery.min.js', false, '3.6', true);
wp_enqueue_script('jquery');
}
}
add_action('template_redirect', 'optimize_jquery');
These are a couple of simple Terminal commands that will help you customize the Dock in OS X v10.9 (Mavericks).
To add a space in between icons or sets of icons, just run this command a few times and it will add "blank spaces" at the end of the dock. From there, you can simply drag and drop the spaces to any location on the dock, thus creating groups:
defaults write com.apple.dock persistent-apps -array-add '{"tile-type"="spacer-tile”;}’ && killall Dock
If you like to hide the dock, but the delay to unhide it drives you crazy, this will make it pop out instantly:
defaults write com.apple.dock autohide-delay -float 0 && killall Dock
#Add Support for SVG
AddType image/svg+xml svg
AddType image/svg+xml .svg
AddType image/svg+xml svgz
#Enable Compression
AddOutputFilterByType DEFLATE image/svg+xml
#Enable Caching
<FilesMatch "\.(jpg|jpeg|png|gif|swf|svg)$">
Header set Cache-Control "max-age=604800, public"
<img src="images/my-image.svg" alt="My Image" />
<svg version="1.1" xmlns="http://www.w3.org/2000/svg" xmlns:xlink="http://www.w3.org/1999/xlink">
<symbol id="icon-star-full" viewBox="0 0 16 16">
<title>star-full</title>
<path class="path1" d="M16 6.204l-5.528-0.803-2.472-5.009-2.472 5.009-5.528 0.803 4 3.899-0.944 5.505 4.944-2.599 4.944 2.599-0.944-5.505 4-3.899z"></path>
</symbol>
<symbol id="icon-check" viewBox="0 0 1024 1024">
<title>check</title>
<path class="path1" d="M69.143 530.286q0-22.857 16-38.857l77.714-77.714q16-16 38.857-16t38.857 16l168 168.571 374.857-375.429q16-16 38.857-16t38.857 16l77.714 77.714q16 16 16 38.857t-16 38.857l-491.429 491.429q-16 16-38.857 16t-38.857-16l-284.571-284.571q-16-16-16-38.857z"></path>
</symbol>
</svg>
<style type="text/css">
.svg-sprite {display: none;}
</style>
<script type="text/javascript">
// Ajax in SVGs
var ajax = new XMLHttpRequest();
ajax.open("GET", "/includes/icons.svg", true);
ajax.send();
ajax.onload = function(e) {
var div = document.createElement("div");
div.className = "svg-sprite";
div.innerHTML = ajax.responseText;
document.body.insertBefore(div, document.body.firstChild);
}
</script>
<script type="text/javascript">
!function(a,b){"function"==typeof define&&define.amd?define([],function(){return a.svg4everybody=b()}):"object"==typeof exports?module.exports=b():a.svg4everybody=b()}(this,function(){/*! svg4everybody v2.0.0 | github.com/jonathantneal/svg4everybody */
function a(a,b){if(b){var c=!a.getAttribute("viewBox")&&b.getAttribute("viewBox"),d=document.createDocumentFragment(),e=b.cloneNode(!0);for(c&&a.setAttribute("viewBox",c);e.childNodes.length;)d.appendChild(e.firstChild);a.appendChild(d)}}function b(b){b.onreadystatechange=function(){if(4===b.readyState){var c=document.createElement("x");c.innerHTML=b.responseText,b.s.splice(0).map(function(b){a(b[0],c.querySelector("#"+b[1].replace(/(\W)/g,"\\$1")))})}},b.onreadystatechange()}function c(c){function d(){for(var c;c=e[0];){var j=c.parentNode;if(j&&/svg/i.test(j.nodeName)){var k=c.getAttribute("xlink:href");if(f&&(!g||g(k,j,c))){var l=k.split("#"),m=l[0],n=l[1];if(j.removeChild(c),m.length){var o=i[m]=i[m]||new XMLHttpRequest;o.s||(o.s=[],o.open("GET",m),o.send()),o.s.push([j,n]),b(o)}else a(j,document.getElementById(n))}}}h(d,17)}c=c||{};var e=document.getElementsByTagName("use"),f="polyfill"in c?c.polyfill:/\bEdge\/12\b|\bTrident\/[567]\b|\bVersion\/7.0 Safari\b/.test(navigator.userAgent)||(navigator.userAgent.match(/AppleWebKit\/(\d+)/)||[])[1]<537,g=c.validate,h=window.requestAnimationFrame||setTimeout,i={};f&&d()}return c});
</script>
// ************************************
// Enable SVG Uploads
// ************************************
add_filter('upload_mimes', 'custom_upload_mimes');
function custom_upload_mimes ( $existing_mimes=array() ) {
$existing_mimes['svg'] = 'mime/type';
return $existing_mimes;
}
<svg><use xlink:href="#icon-check"></use></svg>
OR...
<img src="images/my-image.svg" alt="My Image" />
While you are welcome to buy paperback/hardback copies of your textbooks, we recommend renting the eBook/pdf version of the books as they are much more affordable. You can find all the books for your courses here:
DMDC 1012 - PDF Available in Canvas